Part 3 : Detecting Touch in TFT LCD Display using Library
- electronicseternit
- Dec 31, 2021
- 2 min read
Updated: Jan 2, 2022
In this tutorial, we will be learning how to detect touch in a TFT LCD Display.
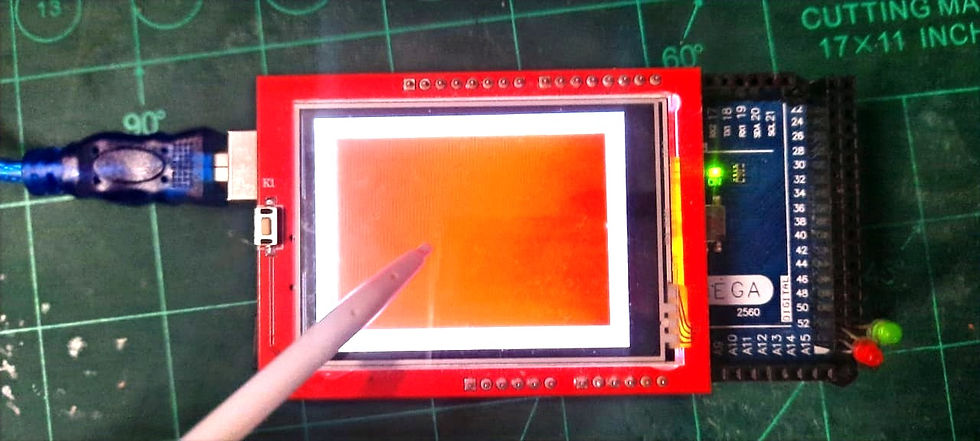
The code to detect touch is quite simple as shown below :
#include <SPFD5408_Adafruit_GFX.h> // Core graphics library
#include <SPFD5408_Adafruit_TFTLCD.h> // Hardware-specific library
#include <SPFD5408_TouchScreen.h>
#define LCD_CS A3 // Chip Select goes to Analog 3
#define LCD_CD A2 // Command/Data goes to Analog 2
#define LCD_WR A1 // LCD Write goes to Analog 1
#define LCD_RD A0 // LCD Read goes to Analog 0
#define LCD_RESET A4 // Can alternately just connect to Arduino's reset pin
#define BLACK 0x0000
#define BLUE 0x001F
#define RED 0xF800
#define GREEN 0x07E0
#define CYAN 0x07FF
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
//These are the pins for the shield!
#define YP A1
#define XM A2
#define YM 7
#define XP 6
Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET);
//Adjust the sensitivity of the touchscreen to get desired output
#define sensitivity 1000
TouchScreen ts = TouchScreen(XP, YP, XM, YM, sensitivity);
// Calibrates value
#define MINPRESSURE 10
#define MAXPRESSURE 2000
void setup(void) {
Serial.begin(9600);
tft.reset();
tft.begin(0x9341);
tft.setRotation(3);
tft.fillScreen(WHITE);
}
void loop() {
TSPoint pinitial;
pinMode(XM, OUTPUT); //Pin Config for TouchScreen
pinMode(YP, INPUT); //Pin Config for TouchScreen
pinitial = ts.getPoint();
pinMode(XM, OUTPUT); //Pin Config for LCD Display
pinMode(YP, OUTPUT); //Pin Config for LCD Display
while (pinitial.z > MINPRESSURE && pinitial.z < MAXPRESSURE) {
tft.fillRect(20,20,280,200,GREEN);
delay(500);
Serial.print("pinitial.x : ");
Serial.println(pinitial.x);
tft.fillRect(20,20,280,200,RED);
break;
}
}
The below PIN configurations are necessary when detecting touch
pinMode(XM, OUTPUT); //Pin Config for TouchScreen
pinMode(YP, INPUT); //Pin Config for TouchScreen
pinitial = ts.getPoint();
In contrast, when we are changing the graphical output in the TFT Display, we need to set pin configuration as shown below :
pinMode(XM, OUTPUT); //Pin Config for LCD Display
pinMode(YP, OUTPUT); //Pin Config for LCD Display
However, it is important to understand that the above pins arrangement are subjected to experimentation. Different LCD Displays purchased from different supplier and companies may behave in a different and hence please try out a few pins configuration of OUTPUT and INPUT to get the desired result.
For more detailed instructions, please check my YouTube video below :
Commenti