In this tutorial, we will be looking at how to identify the coordinate of a point being touch in the LCD Display. Again, for this tutorial, we are using SPFD_4058 library.
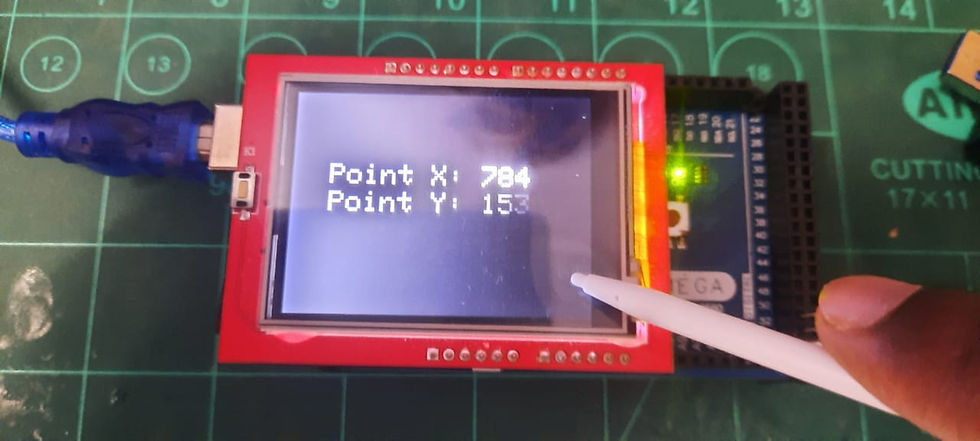
Identifying a touch point coordinate will help us to create an interface and even trigger a particular output reaction thru GPIO pins. Hence, it is important to identify coordinates of a touch point in a LCD Display.
Below is the code used for this tutorial :
#include <SPFD5408_Adafruit_GFX.h>
#include <SPFD5408_Adafruit_TFTLCD.h>
#include <SPFD5408_TouchScreen.h>
#define LCD_CS A3
#define LCD_CD A2
#define LCD_WR A1
#define LCD_RD A0
#define LCD_RST A4
#define BLACK 0x0000
#define BLUE 0x001F
#define RED 0xF800
#define GREEN 0x07E0
#define CYAN 0x07FF
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
//Touch Screen Pins for LCD Display
#define YP A1
#define XM A2
#define YM 7
#define XP 6
// Calibrates touchscreen value
#define SENSITIVITY 300
#define MINPRESSURE 10
#define MAXPRESSURE 1000
Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RST);
TouchScreen ts = TouchScreen(XP, YP, XM, YM, SENSITIVITY);
void setup(void) {
Serial.begin(9600);
tft.reset();
tft.begin(0x9341);
tft.setRotation(3);
tft.fillScreen(BLACK);
}
void loop() {
TSPoint p;
p = ts.getPoint();
pinMode(XM, OUTPUT); //Pins configures again for TFT control
pinMode(YP, OUTPUT);
tft.setCursor(40, 80);
tft.setTextSize(3);
tft.setTextColor(WHITE);
tft.print("Point X: ");
tft.println(p.x);
tft.setCursor(40, 110);
tft.print("Point Y: ");
tft.println(p.y);
delay(200);
tft.fillRect(180,40,100,100,BLACK);
}
You can adjust the sensibility of your touch screen by changing the value as shown below :
#define SENSITIVITY 300
TouchScreen ts = TouchScreen(XP, YP, XM, YM, SENSITIVITY);
Different LCD Display may have different sensitivity, hence experiment this by changing the value "300" to some other values depending on the sensitivity that you want.
For more detailed explanation, feel free to check my YouTube below :
コメント