Part 1 : LCD Display using Library
- electronicseternit
- Nov 15, 2021
- 3 min read
Updated: Dec 27, 2021
In this tutorial, we will be looking at how to use 2.4” Thin Film Transistor LCD 240RGB x 320 Resolution and 262K color.
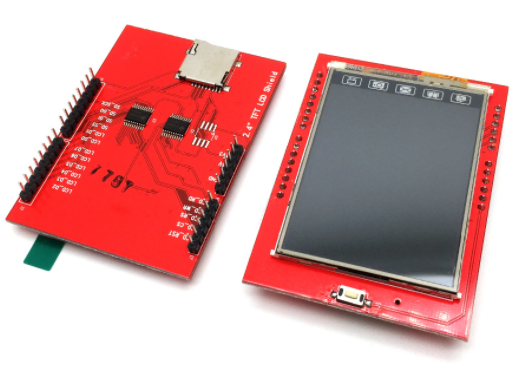
The above shield can be easily topped over an Arduino UNO. Hence, there is no need to bother about pin arrangements or circuit setup. Just be gentle when connecting the LCD Display to Arduino to avoid breaking the pins of the LCD.
Figure below shows the pinout of the LCD module when connected to an Arduino UNO. The numbered pin boxes located outside of the figure shows the respective Arduino Pins when the shield connected is stacked on top of Arduino Uno.
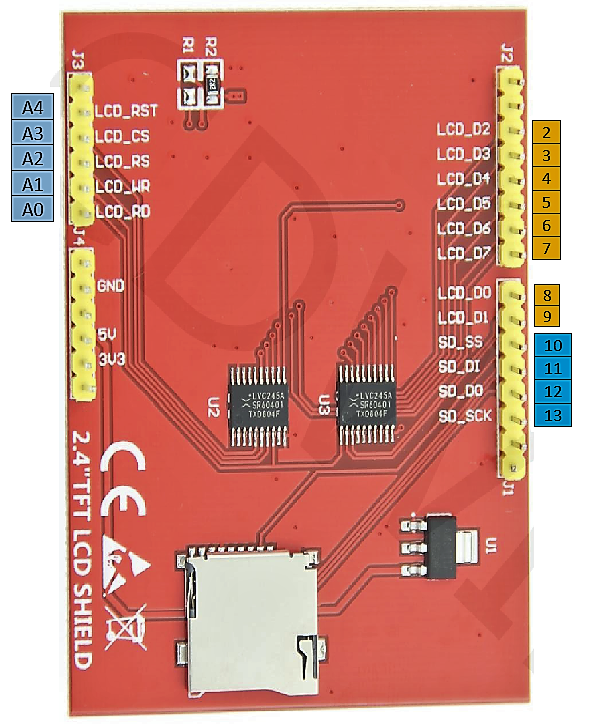
The table below shows the function of LCD_RST, CS, RS, WR and RD pins which are crucial in determining the behavior of LCD Display.
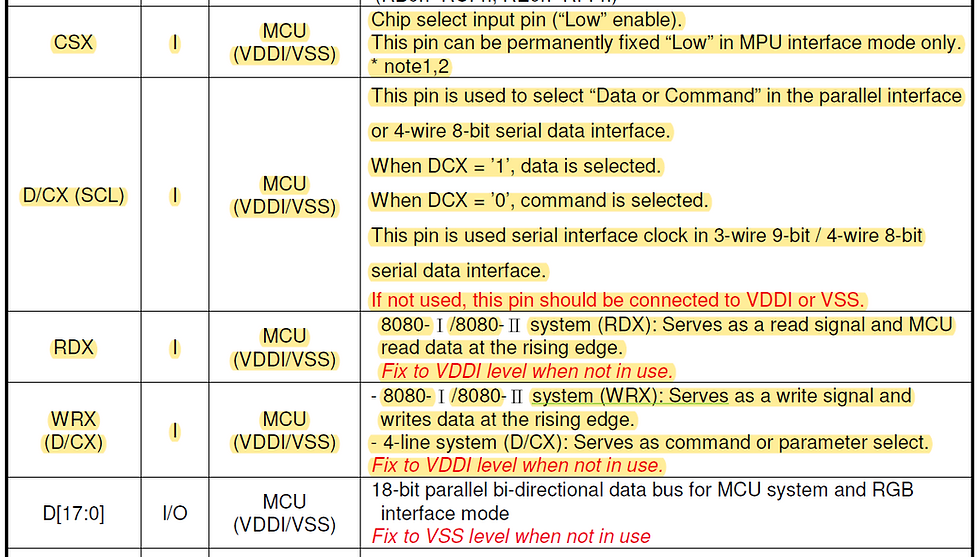
D/CX pin in table above is equivalent to RS pin from the LCD. D[17:0] refers to the Data Pin. DataPins from LCD Module refers to pin LCD_D0 to LCD_D7 which are 8 pins in total. These pins are connected to Arduino Digital Pin 2 – 9. For this tutorial, we are not utilizing the SD Card pins.
For this tutorial, we will be using SPFD5408 Library which is available on the net. You can download the library and manuals from here. All credit goes to the content creator in this website for creating this library.
Below is the code used in this tutorial.
#include <SPFD5408_Adafruit_GFX.h>
#include <SPFD5408_Adafruit_TFTLCD.h>
#include <SPFD5408_TouchScreen.h>
#define LCD_CS A3
#define LCD_CD A2
#define LCD_WR A1
#define LCD_RD A0
#define LCD_RST A4
#define BLACK 0x0000
#define BLUE 0x001F
#define RED 0xF800
#define GREEN 0x07E0
#define CYAN 0x07FF
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RST);
void setup(void) {
Serial.begin(9600);
uint16_t identifier = tft.readID();
Serial.print("Controller ID : ");
Serial.println(identifier,HEX);
tft.reset();
tft.begin(0x9341);
tft.setRotation(0);
tft.fillScreen(BLACK);
tft.fillScreen(RED);
tft.fillScreen(BLUE);
tft.fillScreen(GREEN);
tft.fillScreen(BLACK);
tft.setRotation(3);
tft.setCursor(10, 5);
tft.setTextColor(GREEN);
tft.setTextSize(2);
tft.println("Electronics");
tft.setCursor(10, 20);
tft.setTextColor(GREEN);
tft.setTextSize(2);
tft.println("Eternity");
tft.setCursor(10, 37);
tft.setTextColor(RED);
tft.setTextSize(2.5);
tft.println("13/4/2021");
tft.setCursor(10, 54);
tft.setTextColor(WHITE);
tft.setTextSize(2.5);
tft.print("ID :");
tft.print(identifier, HEX);
int w = tft.width();
int h = tft.height();
//drawLine(x1, y1, x2, y2, color);
//draw VerticalLine
tft.drawLine(w/2, 0, w/2, h, YELLOW);
//draw HorizontalLine
tft.drawLine(0, h/2, w, h/2, YELLOW);
//drawRectangle(x1, y1, width, height, color);
tft.drawRect((w/2)+10, 10, 40, 30, BLUE);
//tft.fillRect(x1, y1, width, height, color1);
tft.fillRect((w/2)+10, 50, 40, 30, BLUE);
//tft.drawCircle(x, y, radius, color);
tft.drawCircle((w/2)+80, 25, 15, YELLOW);
//tft.fillCircle(x, y, radius, color);
tft.fillCircle((w/2)+80, 65, 15, YELLOW);
//tft.drawTriangle(peak_x1, peak_y1,leftx2, lefty2, rightx3, righty3, color);
//tft.color565(red, green, blue) each color goes up to 255
tft.drawTriangle(30, 130, 10, 170, 50, 170, tft.color565(255, 0, 0));
//tft.fillTriangle(peak_x1, peak_y1,leftx2, lefty2, rightx3, righty3, color);
tft.fillTriangle(30, 180, 10, 220, 50, 220, tft.color565(0, 255, 0));
//tft.drawRoundRect(x, y, width, height, curvature, color);
tft.drawRoundRect(70, 130, 40, 40, 5, tft.color565(0, 0, 255));
//tft.fillRoundRect(x, y, width, height, curvature, color);
tft.fillRoundRect(70, 180, 40, 40, 5, tft.color565(0, 0, 255));
}
void loop(){
}
I have placed all my commands in the void setup as I only need it to run once. Try running the commands one by one for each commands for better understanding. You can do so by commenting out the rest of the commands to observe a single command at a time.
Another important point to consider, when using an existing library is that you need to find a library that works well with your LCD Display. Reason being is the same 2.4" TFT LCD Display may have different display and touch screen controllers which makes only specific library usable with a particular LCD Display. Hence, buying LCD Display from a reliable provider would help in identifying controllers related to a LCD Display and the respective library that works with it.
Good Luck Trying. For more detailed explanation, check out my YouTube video below :
Comments