In this tutorial, we will be looking on how to create a Simple Wifi Server using Static IP and a bit of CSS Formatting to accentuate the looks of our Wifi Server.
Without further a do, below is the code that will be used in this tutorial :
#include <WiFi.h>
const char* ssid = "SSID_Name";
const char* password = "Wifi Password";
WiFiServer server(80);
void setup()
{
Serial.begin(115200);
pinMode(5, OUTPUT); // set the LED pin mode
delay(10);
Serial.println();
Serial.println();
Serial.print("Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
// Set your Static IP address
IPAddress local_IP(192, 168, 68, 222);
// Set your Gateway IP address
IPAddress gateway(192, 168, 68, 1);
IPAddress subnet(255, 255, 0, 0);
IPAddress primaryDNS(8, 8, 8, 8); // optional
IPAddress secondaryDNS(8, 8, 4, 4); // optional
if (!WiFi.config(local_IP, gateway, subnet, primaryDNS, secondaryDNS)) {
Serial.println("STA Failed to configure");
}
Serial.print("Customized IP: ");
Serial.println(WiFi.localIP());
server.begin();
}
int value = 0;
void loop() {
WiFiClient client = server.available();
if (client) {
Serial.println("New Client.");
String currentLine = "";
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
if (c == '\n') {
// if the current line is blank, you got two newline characters in a row.
// that's the end of the client HTTP request, so send a response:
if (currentLine.length() == 0) {
// HTTP headers always start with a response code (e.g. +)
// and a content-type so the client knows what's coming, then a blank line:
client.println("HTTP/1.1 200 OK");
client.println("Content-type:text/html");
client.println();
//The HTTP 200 OK success status response code indicates that the request has succeeded
//A 200 response is cacheable by default.
client.print("<head><style>.button{background-color:#4CAF50;border:none;color:white;padding:15px 32px;text-align:center;text-decoration:none;display:inline-block;font-size:16px;margin:4px 2px;cursor:pointer;}.button1{background-color:#2ECC71;}.button2{background-color:#E74C3C;margin-left:10px;}</style></head>");
client.print("<h1 style='color:#34495E'>Electronics Eternity</h1>");
client.print("<a href=\"H\"class='button button1'>Turn ON LED</a>");
client.print("<a href=\"L\"class='button button2'>Turn OFF LED</a>");
client.println();
break;
}
else { // if you got a newline, then clear currentLine:
currentLine = "";
}
}
else if (c != '\r') { // if you got anything else but a carriage return character,
currentLine += c; // add it to the end of the currentLine
}
// Check to see if the client request was "GET /H" or "GET /L":
if (currentLine.endsWith("GET /H")) {
digitalWrite(5, HIGH); // GET /H turns the LED on
}
if (currentLine.endsWith("GET /L")) {
digitalWrite(5, LOW); // GET /L turns the LED off
}
}
}
// close the connection:
client.stop();
Serial.println("Client Disconnected.");
}
}
I have covered how to create a customized Static IP in the previous tutorial, hence I won't be explaining this in this tutorial.
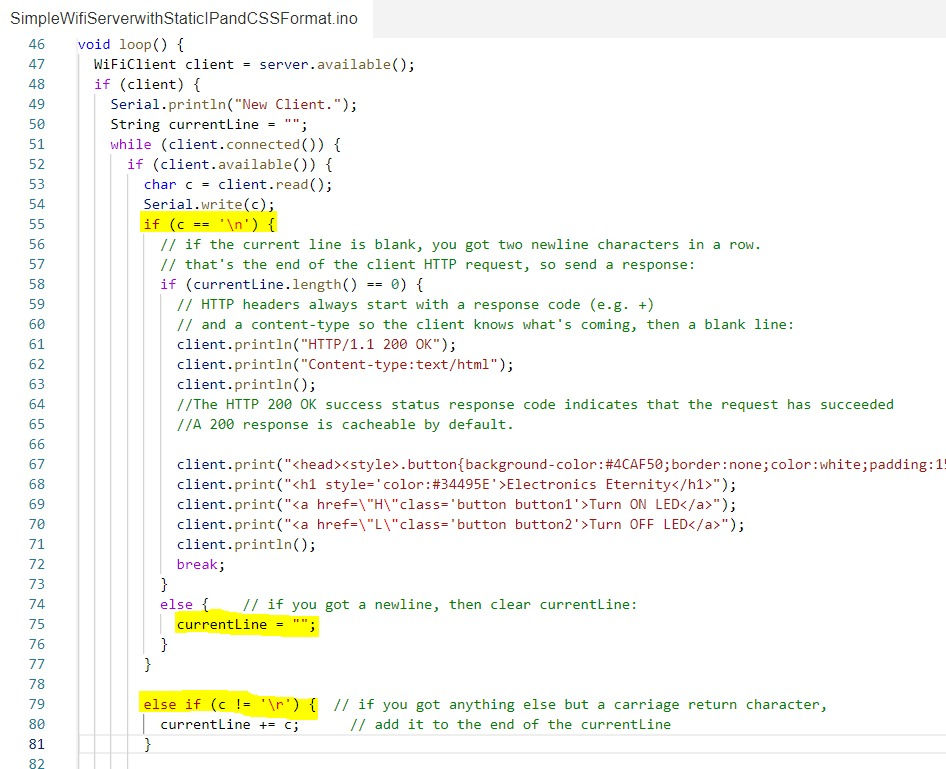
Now, let's take a look at the above part of the code. The main processing happens in this portion. Logically, the above code looks for 3 items when a client is connected to the Wifi Server which are (c == '\n') { ...}, currentLine = ""; and else if (c != '\r').
When (c == '\n') { ...}, we will be basically printing the website. We will discuss in detail on how the webpage is created in the later part of this tutorial. We also check there is any content on the webpage by using if (currentLine.length() == 0) {....}. The program will only create the webpage when the currentLine equals to 0 and do nothing is not equal to 0. Up until this part, we have covered on the creation of the website.
However, when a "\n" is not found in the website and currentLine is not equal to, the program then stores whatever array or strings into currentLine at else if (c != '\r'). By storing these characters or responses from the server, we can decide on how we can act on it in which I find it very effective.
We have looked into the logic that creates the website and also how to store certain response from the server. Accordingly, let's look at what we do with the responses.
if (currentLine.endsWith("GET /H")) {
digitalWrite(5, HIGH); // GET /H turns the LED on
}
if (currentLine.endsWith("GET /L")) {
digitalWrite(5, LOW); // GET /L turns the LED off
}
When "/H" is received from the Wifi Server, the LED is turned ON and when "/L" is received, the LED is turned OFF. At this point, you may be wondering where does the "/H" and "/L" comes from. Without causing any further distress, I will reveal it down to you. These returns are created from the HTML code that we use to create the website which we will discuss in detail as follows.
client.print("<head><style>.button{background-color:#4CAF50;border:none;color:white;padding:15px 32px;text-align:center;text-decoration:none;display:inline-block;font-size:16px;margin:4px 2px;cursor:pointer;}.button1{background-color:#2ECC71;}.button2{background-color:#E74C3C;margin-left:10px;}</style></head>");
client.print("<h1 style='color:#34495E'>Electronics Eternity</h1>");
client.print("<a href=\"H\"class='button button1'>Turn ON LED</a>");
client.print("<a href=\"L\"class='button button2'>Turn OFF LED</a>");
client.println();
The above contains the HTML code used. If we take out "client.print(" and other Arduino syntaxes, we simply have the workable HTML code which would look something like below :
<head><style>.button{background-color:#4CAF50;border:none;color:white;padding:15px 32px;text-align:center;text-decoration:none;display:inline-block;font-size:16px;margin:4px 2px;cursor:pointer;}.button1{background-color:#2ECC71;}.button2{background-color:#E74C3C;margin-left:10px;}
</style>
</head>
<h1 style='color:#34495E'>Electronics Eternity</h1>
<a href=\"H\"class='button button1'>Turn ON LED</a>
<a href=\"L\"class='button button2'>Turn OFF LED</a>
You can take the exact code above, copy-paste into a notepad and save it as a .html file, it will show you how the website looks like once you open the file in any browser such as Chrome, MozilaFireFox, Opera and etc.
By looking at the HTML code alone, you will be able to understand better on how the webpage is created.
The webpage looks like below. Note the differences in the url when TURN ON or TURN OFF button is clicked.

The URL ends with 'H'
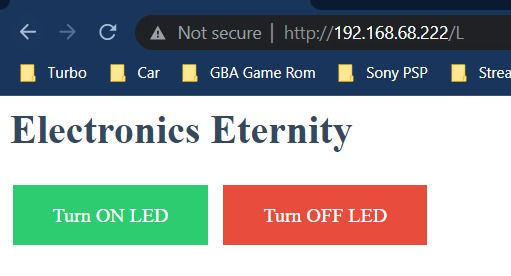
The URL ends with 'L'
Based on the differences of URL end character, the program can decide what action can be done as shown below.
// Check to see if the client request was "GET /H" or "GET /L":
if (currentLine.endsWith("GET /H")) {
digitalWrite(5, HIGH); // GET /H turns the LED on
}
if (currentLine.endsWith("GET /L")) {
digitalWrite(5, LOW); // GET /L turns the LED off
}
When 'H' is identified, the program will write pin5 as HIGH where we have attached LED to it whereas when 'L' is identified, the program will write pin5 as LOW.
With that, we have come to the end of the tutorial. I hope you find this useful.
For more detailed instruction on this, feel free to check out my YouTube video :
Comments