Gas Leakage Detection System
- electronicseternit
- Sep 29, 2020
- 2 min read
In this tutorial, we will be learning how to create a complete Gas Leakage Detection System by using Arduino, MQ5 Sensor and a GSM Module. I will be using SIM900A Mini GSM Module.
Before you start with this tutorial, please ensure if you have gone thru other GSM and MQ5 basics tutorial to help understand this tutorial better.
Part 1 : GSM Setup
GSM : Part 2 (Controlling GSM using Arduino's Serial Monitor) : https://electronicseternit.wixsite.com/electronicsforlife/post/gsm-part-2-controlling-gsm-using-arduino-s-serial-monitor
Using MQ5 Gas Sensor with Arduino :
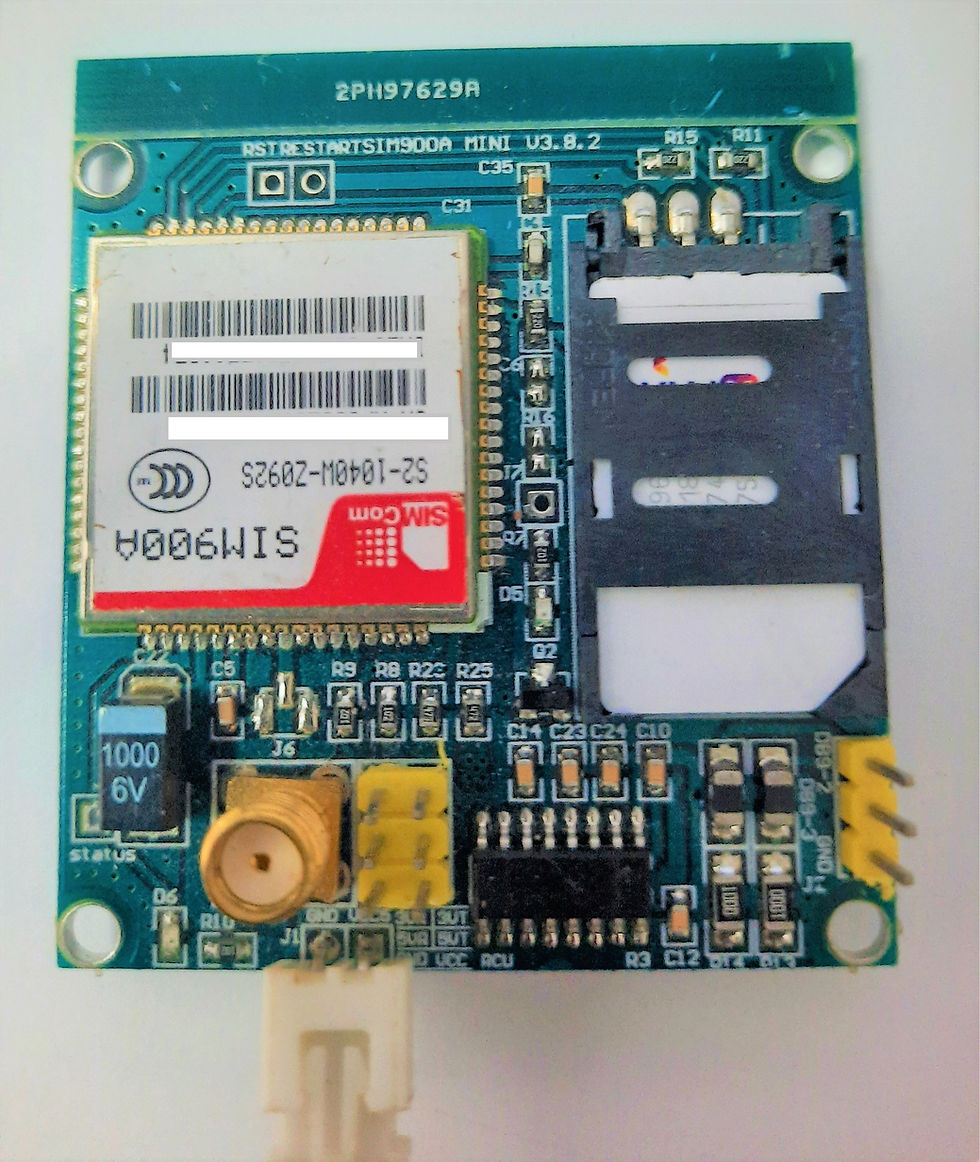
The referred components will be connected as shown in Figure below. It is important to ensure all the components are correctly connected as shown.
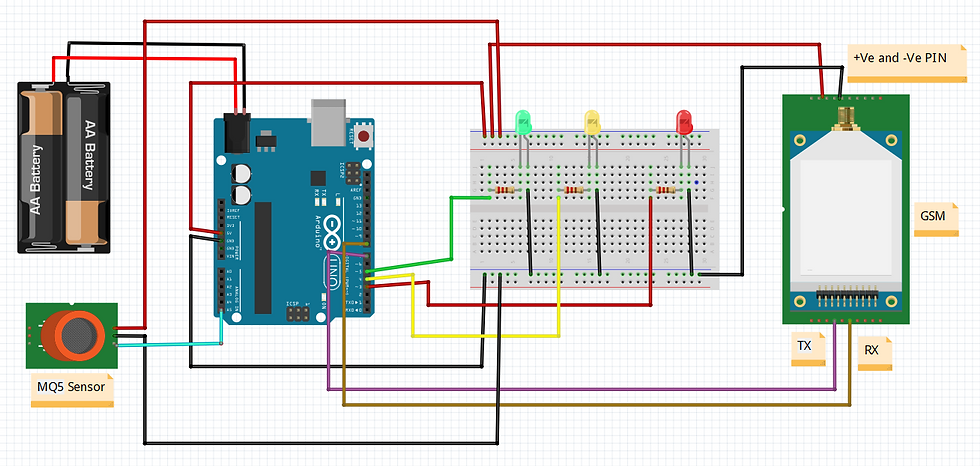
Once the circuit has been setup, you can then upload the below code to complete your Gas Leakage Detection system.
#include <SoftwareSerial.h>
SoftwareSerial SIM900A(7, 8);
int redLed = 5;
int yellowLed = 4;
int greenLed = 3;
int smokeLevel = A5;
int threVal = 250;
String gsmmsg;
void setup() {
// put your setup code here, to run once:
SIM900A.begin(9600); // Setting the baud rate of GSM Module
Serial.begin(9600); // Setting the baud rate of Serial Monitor (Arduino)
pinMode(smokeLevel,INPUT);
pinMode(redLed, OUTPUT);
pinMode(yellowLed, OUTPUT);
pinMode(greenLed, OUTPUT);
delay(100);
Serial.println("SIM900A Ready");
//test if AT Command is OK
SIM900A.println("AT");
delay(1000);
gsmmsg = SIM900A.readString();
if (gsmmsg.indexOf('OK') >= 1) {
Serial.println("AT Command is OK");
digitalWrite(redLed, HIGH);
//Serial.println("RED LED TURNED ON");
}
else
{Serial.println("AT NOT OK");
};
gsmmsg = "";
//Get Power Value for the GSM
SIM900A.println("AT+CBC");
delay(1000);
String CBC = SIM900A.readString();
int firstcomma = CBC.indexOf(',');
int secondcomma = CBC.indexOf(',', firstcomma + 1);
String battery = CBC.substring(firstcomma + 1, secondcomma);
int charge = battery.toInt();
if (charge >= 50) {
digitalWrite(yellowLed, HIGH);
}
String newcharge = String(charge); // int must be changed to String before it can be concatenated
String result = "Power is at (" + newcharge + "/100)";
Serial.println(result);
gsmmsg = "";
//Set GSM to SMS Mode
SIM900A.println("AT+CMGF=1");
delay(1000);
String gsmmsg = SIM900A.readString();
if (gsmmsg.indexOf('OK') >= 1) {-
Serial.println("Succesfully Set GSM to SMS Message Format!");
digitalWrite(greenLed, HIGH);
//Serial.println("GREEN LED TURNED ON");
}
else
{
Serial.println("Set GSM to SMS Message Format Failed");
};
//Creating Identifier for GSM
SIM900A.println("AT+CGMI");
delay(1000);
String modelarray = SIM900A.readString();
int ok = modelarray.indexOf('OK',1);
String gsmmodel = modelarray.substring(0, ok-1);
gsmmodel.replace("\n", "");
SIM900A.println("AT+CGSN");
String imeiarray = SIM900A.readString();
delay(1000);
int ok2 = imeiarray.indexOf('OK', 1);
String imeinumber = imeiarray.substring(0, ok2-1);
imeinumber.replace("\n", "");
String identifier = gsmmodel + " IMEI:" + imeinumber;
Serial.println(identifier);
//Sending Initialization Message
String initialize = "GSM is ready | " + result + " |" + identifier;
SIM900A.println("AT+CMGF=1");
delay(1000);
SIM900A.println("AT+CMGS=\"+601XXXXXXXXX\"\r");
delay(1000);
SIM900A.println(initialize);
delay(100);
SIM900A.println((char)26);
Serial.println("Initialization Message Sent");
gsmmsg = "";
}
void loop() {
int sensorVal = analogRead(smokeLevel);
Serial.println(sensorVal);
if(sensorVal > threVal){
String Output = "Gas Leakage Detected (";
Output += String(sensorVal);
Output += "/1024)";
Serial.println(Output);
//Sending Alert Message
SIM900A.println("AT+CMGF=1");
delay(1000);
SIM900A.println("AT+CMGS=\"+601XXXXXXXXX\"\r");
delay(1000);
SIM900A.println(Output);
delay(100);
SIM900A.println((char)26);
Serial.println("Initialization Message Sent");
}
}
For more information, you can refer my video below.
Good Luck Trying!
تعليقات